Supercharge lists in Python: bisect
stdlib, python
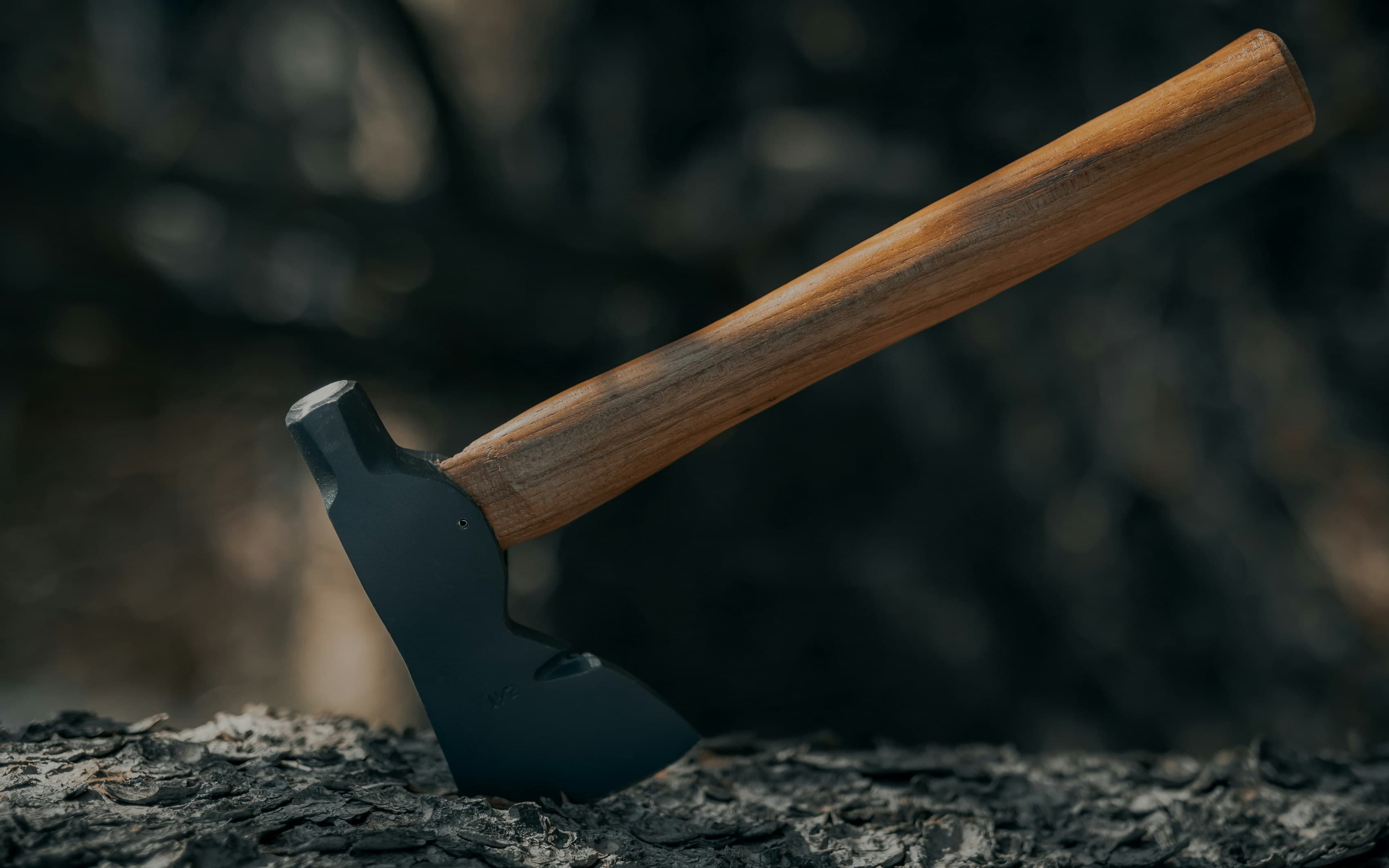
Welcome to the first part of my advanced Python series! In this blog post, we'll dive into the bisect
module, a powerful tool in Python's standard library that can supercharge your list manipulation skills.
What is Bisection?
Bisection is an algorithm that employs a simple yet effective strategy: "cut the thing in half until you find the right point." While it may sound basic, its applications are far-reaching and can greatly enhance your coding toolkit, especially when dealing with sorted lists.
Some practical uses of bisection include:
- Efficiently Sorting Lists: Keeping a list sorted while adding new items.
- Binary Search: A classic algorithm for finding elements in a sorted list.
- Merging Sorted Items: Combining multiple sorted lists into one.
These foundational algorithms can be further leveraged in more complex, real-world scenarios, such as the "Merging Sorted Lists" problem on LeetCode.
The bisect
Module in Action
The bisect
module in Python provides a range of functions to implement bisection algorithms efficiently. Let's explore one of its key functions, bisect.insort()
, through a practical example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
import bisect # Test cases for merging sorted lists test_cases = [ ([[1, 4, 5], [1, 3, 4], [2, 6]], [1, 1, 2, 3, 4, 4, 5, 6]), ([[3, 4, 5, 6, 7], [0, 1, 2], [8, 9]], [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]), # ... (more test cases) ] def mergeKLists(lists: list[list[int]]) -> list[int]: s = [] for _list in lists: for _el in _list: bisect.insort(s, _el) return s # Run tests for _in, _out in test_cases: assert mergeKLists(_in) == _out
In the above code, we define a mergeKLists
function that takes a list of sorted lists (lists
) and returns a single merged, sorted list. The bisect.insort()
function is used to efficiently insert elements into the s
list while maintaining its sorted order.
Exploring bisect.bisect()
One of the most powerful functions in the bisect
module is bisect.bisect()
. Let's take a closer look at its documentation:
Return the index where to insert item x in list a, assuming a is sorted.The return value i is such that all e in a[:i] have e <= x, and all e in a[i:] have e > x. So if x already appears in the list, a.insert(i, x) will insert just after the rightmost x already there.Optional args lo (default 0) and hi (default len(a)) bound the slice of a to be searched.A custom key function can be supplied to customize the sort order.
This function is particularly useful in divide-and-conquer algorithms, allowing you to efficiently find the correct position to insert an element into a sorted list.
Conclusion
The bisect
module is a hidden gem in Python's standard library, offering a range of powerful tools for list manipulation. By leveraging bisection algorithms, you can write more efficient and elegant code, especially when working with sorted data.
Stay tuned for the final part of our advanced Python series, where we'll explore another exciting module and its applications!
Happy coding!