MagicMock and side_effect tricks
testing, python
The side_effect attribute of MagicMock in Python allows you to customize the return values or behaviors of mocked methods, enabling dynamic responses such as varying return values, custom logic, or exception raising across multiple calls.
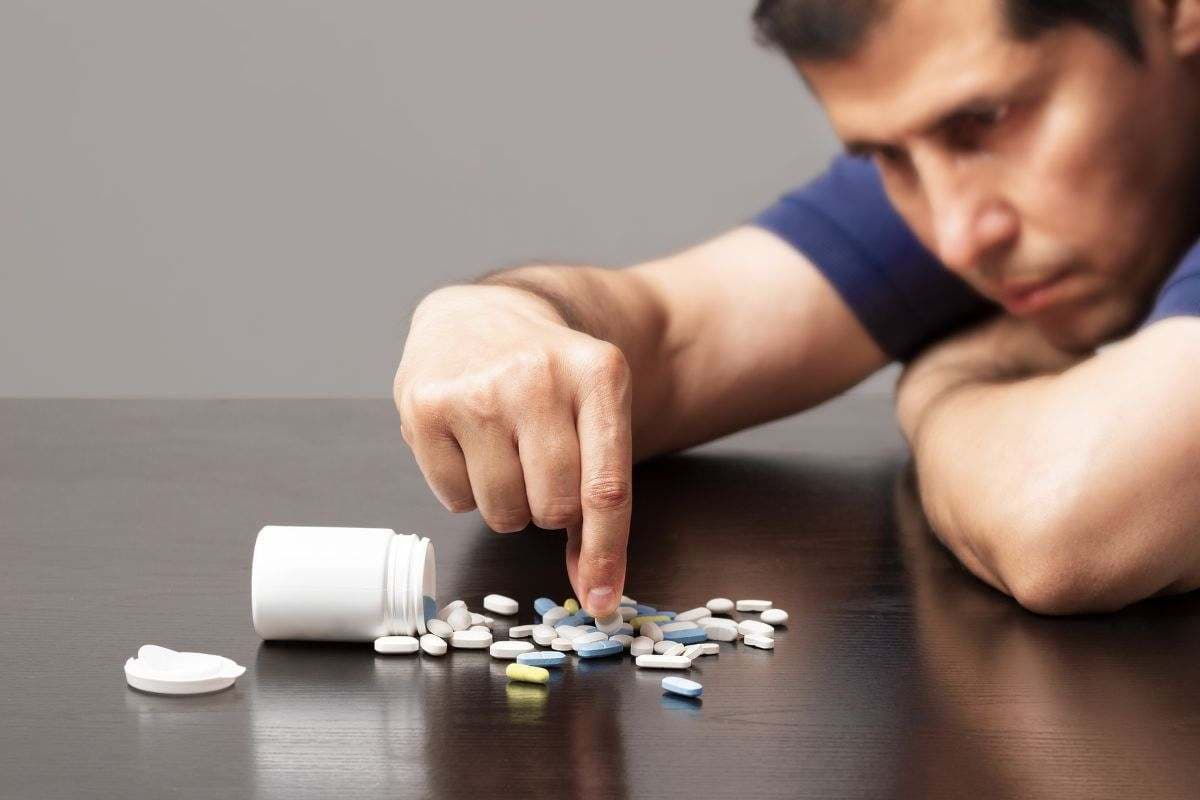
Using MagicMock
Side Effect for Custom Mock Behaviors in Python
When writing unit tests in Python, you often need to mock objects or functions to isolate the code you're testing. The unittest.mock
module provides the MagicMock
class, which allows you to create mock objects with flexible behaviors. One of the most powerful features of MagicMock
is its side_effect
attribute. This lets you define custom return values or behaviors that change with each call to the mocked method, offering greater control over how the mock behaves across multiple invocations.
For example, imagine you're testing a function that calls an external API. If you want the mock to return different responses on subsequent calls, you can use the side_effect
feature. Setting side_effect
to a list, like [None, (1, 2, 3, 4)]
, will make the mock return None
on the first call, and a tuple (1, 2, 3, 4)
on the second call. This is particularly useful when you need to simulate varying results or test different scenarios within a single test case.
1 2 3 4 5 6 7 8 9 10 11
from unittest.mock import MagicMock # Create the mock object mock_obj = MagicMock() # Set side_effect to return different values on each call mock_obj.execute.side_effect = [None, (1, 2, 3, 4)] # Test the mock behavior print(mock_obj.execute()) # Output: None (first call) print(mock_obj.execute()) # Output: (1, 2, 3, 4) (second call)
In this example, the mock's execute()
method returns None
on the first call and a tuple (1, 2, 3, 4)
on the second. Using a list for side_effect
allows you to control the return value on a call-by-call basis.
1 2 3 4 5 6 7 8 9 10 11
from unittest.mock import MagicMock # Create the mock object mock_obj = MagicMock() # Set side_effect to a lambda function mock_obj.execute.side_effect = lambda x: x * 2 # Test the mock with custom logic print(mock_obj.execute(5)) # Output: 10 (5 * 2) print(mock_obj.execute(7)) # Output: 14 (7 * 2)
Here, side_effect
is assigned a lambda function, which doubles the input value each time execute()
is called. This provides dynamic behavior where the return value is based on the arguments passed.
1 2 3 4 5 6 7 8 9 10 11 12 13
from unittest.mock import MagicMock # Create the mock object mock_obj = MagicMock() # Set side_effect to raise an exception mock_obj.execute.side_effect = ValueError("An error occurred") # Test the mock behavior with exception raising try: mock_obj.execute() except ValueError as e: print(f"Exception raised: {e}") # Output: Exception raised: An error occurred
In this example, we use side_effect
to raise an exception when execute()
is called. This is useful for testing error handling in your code when interacting with mocked methods or external dependencies.
side_effect
can be used with a callable (such as a function or lambda), which allows for even more dynamic behaviors. This makes it easy to simulate complex logic, like raising exceptions on certain calls, or returning values based on input arguments. The flexibility of side_effect
ensures that your tests are as close to real-world conditions as possible, even when interacting with mocked or simulated components. Whether you're working with simple mocks or need more intricate behavior, MagicMock
's side_effect
is an essential tool in any tester's toolkit.