Database engineers hate this one weird trick
databases, bash
Implementing The World’s Worst Database.
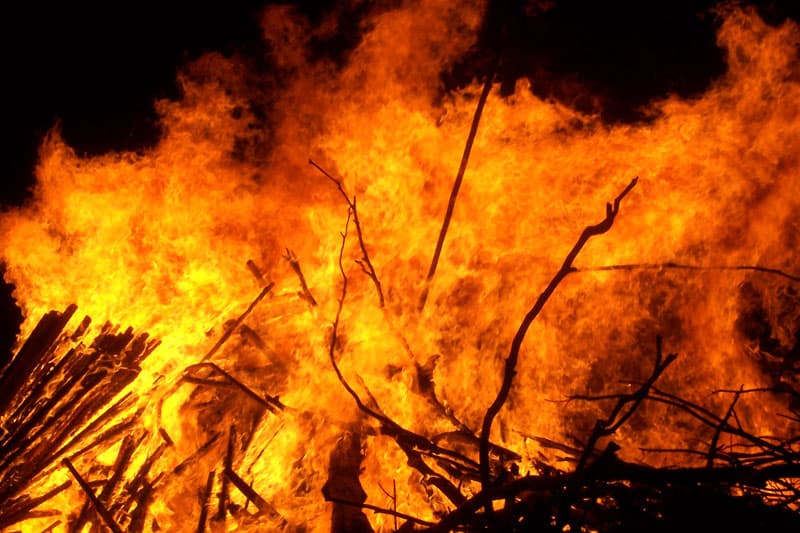
Modern databases seem like magic—until you realize they're just organized files and clever processes. Let's cut through the mystique by building a key-value database in bash. Spoiler: it's shockingly simple... but impractical.
Here's What We're Gonna Do
We'll create four bash functions that handle CRUD operations (Create, Read, Update, Delete). No frameworks, no dependencies—just pure shell magic.
1 2
# Initialize our "database" mkdir -p ~/.database
Heres the reall magic:
1 2 3 4 5 6 7 8 9 10 11
# Create/update a key-value pair dbset() { echo "$2" > ~/.database/"$1"; } # Read a value by key dbget() { cat ~/.database/"$1"; } # Delete a key dbdelete() { rm ~/.database/"$1"; } # Search for keys by pattern dbscan() { ls -l ~/.database | grep "$1"; }
Boom. You've just deployed a database. Let's break it down.
1. How It Works
File-Based Storage
Every "key" is a file in ~/.database
, and its "value" is the file's content. Writing to a key? Just echo text into a file. Reading? Cat the file.
Atomic Operations
Each command runs as a single filesystem operation—no half-written data.
2. ACID Compliance: The Delusional Developer's Checklist
Let's pretend we're enterprise-grade! Here's how our bash DB technically checks ACID boxes:
- Atomicity
Kinda. Eachdbset
ordbdelete
is a single filesystem operation. Your whole value writes or fails—no partial updates. - Consistency
Sure, if you squint. Files either exist with valid content or don't. No constraints? No problem! - Isolation
Single-player mode. The OS handles file locks, but concurrency? Let’s not talk about concurrency. - Durability
As durable as your disk. If your SSD survives, so does your data.
Warning: This is a joke. Real databases handle ACID with transactions, locks, and recovery mechanisms. Don't use this for anything serious.
3. Bonus Round: Hooks and Permissions
Trigger Happy
Want to run code when a key changes? Use inotify-tools
to watch the directory:
1 2 3 4
# Watch for file changes in real-time inotifywait -m ~/.database | while read event; do echo "Database updated! Event: $event" done
Security Features
Permissions? Just use UNIX file permissions. Want to restrict access to a key?
1
chmod 600 ~/.database/my_secret_key # Only you can read/write
4. Why This Is Terrifying
- No backups
- No transactions
- No scalability
- No error handling (What if two processes write at once?)
But hey—it's 4 lines of code.
Conclusion: A Glorious Toy
Congratulations! You've built a "database" that's both awe-inspiring and horrifying. While this isn't replacing PostgreSQL anytime soon, it demystifies core database concepts:
- Data is just organized bytes
- "Queries" are operations on those bytes
- Permissions and hooks are OS-level features
Go forth and experiment — then use a real database for your next project.
Final Warning: If you deploy this, you'll anger the ops gods. You've been warned.